數據庫內容:
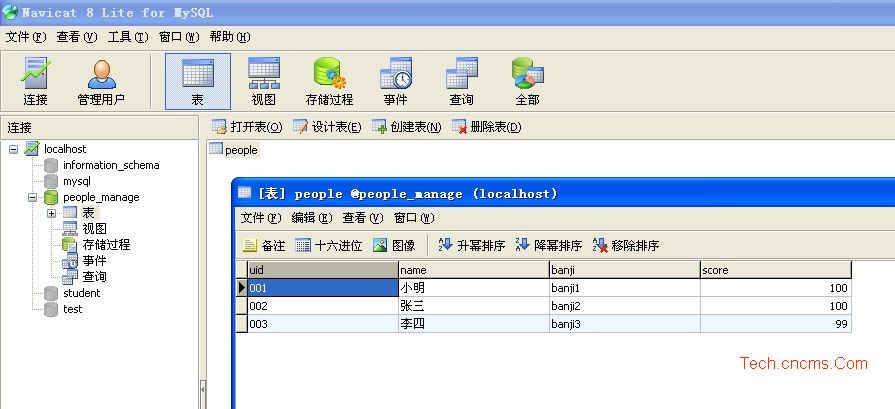
java源代碼:(代碼實現的是查詢成績為100的人員信息,至於其他功能的
代碼中有注釋)
注意:在eclipse裡運行程序的時候,要工程裡插入jar包,否則運行異常!
import java.sql.*;
import java.io.*;
class database_manage {
public Connection conn = null;
public ResultSet rs = null;
private String DatabaseDriver = "com.mysql.jdbc.Driver";
// DataSource 數據源名稱DSN
private String DatabaseConnStr = "jdbc:mysql://localhost:3306/people_manage?useUnicode=true&characterEncoding=utf8"
+ ",root,root";
public void setDatabaseDriver(String Driver) {
this.DatabaseDriver = Driver;
}
public String getDatabaseDriver() {
return (this.DatabaseDriver);
}
public void setDatabaseConnStr(String ConnStr) {
this.DatabaseConnStr = ConnStr;
}
public String getDatabaseConnStr() {
return (this.DatabaseConnStr);
}
public database_manage() {// 構造函數連接數據庫
try {
Class.forName(DatabaseDriver);
} catch (java.lang.ClassNotFoundException e) {
System.err.println("加載驅動器有錯誤:" + e.getMessage());
System.out.print("執行插入有錯誤:" + e.getMessage());// 輸出到客戶端
}
}
public ResultSet query(String sql) {// 查詢數據庫
rs = null;
try {
conn = DriverManager
.getConnection(
"jdbc:mysql://localhost:3306/people_manage?useUnicode=true&characterEncoding=utf8",
"root", "root");
Statement stmt = conn.createStatement();
rs = stmt.executeQuery(sql);
} catch (SQLException ex) {
System.err.println("執行查詢有錯誤:" + ex.getMessage());
System.out.print("執行查詢有錯誤:" + ex.getMessage()); // 輸出到客戶端
}
return rs;
}
public int update_database(String sql) {// 更新或插入數據庫
int num = 0;
try {
conn = DriverManager
.getConnection(
"jdbc:mysql://localhost:3306/people_manage?useUnicode=true&characterEncoding=utf8",
"root", "root");
Statement stmt = conn.createStatement();
num = stmt.executeUpdate(sql);
} catch (SQLException ex) {
System.err.println("執行插入有錯誤:" + ex.getMessage());
System.out.print("執行插入有錯誤:" + ex.getMessage());// 輸出到客戶端
}
CloseDataBase();
return num;
}
public void CloseDataBase() {// 關閉數據庫
try {
conn.close();
} catch (Exception end) {
System.err.println("執行關閉Connection對象有錯誤:" + end.getMessage());
System.out.print("執行執行關閉Connection對象有錯誤:有錯誤:" + end.getMessage()); // 輸出到客戶端
}
}
}
class people {
private String uid;
private String name;
private String banji;
private int score;
public people() {
}
public people(String uid, String name, String banji) {
this.uid = uid;
this.name = name;
this.banji = banji;
}
public people(String uid, String name, String banji, int score) {
this.uid = uid;
this.name = name;
this.banji = banji;
this.score = score;
}
public String getUid() {
return uid;
}
public void setUid(String uid) {
this.uid = uid;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getBanji() {
return banji;
}
public void setBanji(String banji) {
this.banji = banji;
}
public int getScore() {
return score;
}
public void setScore(int score) {
this.score = score;
}
}
public class manage {
private people[] people_array1;// 對象數組
public void add_people(String uid, String name) {
String sql = "insert people (uid,name) values ('" + uid + "','" + name
+ "')";// sql插入語句
// String sql2 = "insert people (uid,name) values ('uid001','tom')";
database_manage db_obj = new database_manage();
db_obj.update_database(sql);
}
public void update_people(String uid, String name) {
String sql = "update people set name='" + name + "' where uid='" + uid
+ "'";
database_manage db_obj = new database_manage();
db_obj.update_database(sql);
}
public void delete_people(String uid) {
String sql = "delete from people where uid='" + uid + "'";
database_manage db_obj = new database_manage();
db_obj.update_database(sql);
}
public people query_people(String uid) {
database_manage db_obj = new database_manage();
// String adminid=null;
String uid_new, name, banji;
uid_new = null;
name = null;
banji = null;
String sql_query = "select * from people where uid='" + uid + "'";
try {
ResultSet rs = db_obj.query(sql_query);
if (rs.next()) {
uid_new = rs.getString("uid");
name = rs.getString("name");
banji = rs.getString("banji");
}
} catch (Exception e) {
e.getMessage();
}
people new_people = new people(uid_new, name, banji);
return new_people;
}
public people[] query_people_byscore(int score) {
database_manage db_obj = new database_manage();
String uid_new, name, banji;
uid_new = null;
name = null;
banji = null;
int score_new = 0;
String sql_query = "select * from people where score=" + score;// sql查詢語句
try {
ResultSet rs = db_obj.query(sql_query);// 查詢後,返回結果集
int num = 0;
ResultSet rs_new = rs;
while (rs_new.next()) {// 統計結果集中學生個數
num++;
}
// System.out.println(num);
people_array1 = new people[num];
int i = 0;
rs.beforeFirst();// 返回結果集的開始
while (rs.next()) {
uid_new = rs.getString("uid");
name = rs.getString("name");
banji = rs.getString("banji");
score_new = rs.getInt("score");
people_array1[i] = new people(uid_new, name, banji, score_new);
i++;
}
} catch (Exception e) {
e.getMessage();
}
return people_array1;
}
public static void main(String args[]) {
/*
* people new_people=new people();
*
* manage mr=new manage(); //mr.add_people("000","小明");插入一個學生的信息
*
* new_people=mr.query_people("001");//查詢uid=001的學生信息,返回對象 System.out.
* println(""+new_people.getName()+" "+new_people.getBanji());
* mr.update_people("004", "小王");更新一個學生的信息
*
* new_people=mr.query_people("004");//更新後查詢
*
* System.out.println(""+new_people.getName()+" "+new_people.getBanji(
* ));
*/
manage mr = new manage();
// mr.delete_people("001");刪除uid=001的學生信息
people[] people_array;// 聲明對象數組
people_array = mr.query_people_byscore(100);// 返回成績為一百的學生類數組,後輸出
int num = 0;
num = people_array.length;
for (int i = 0; i < num; i++) {
System.out.println(people_array[i].getUid() + " "
+ people_array[i].getName() + " "
+ people_array[i].getBanji() + " "
+ people_array[i].getScore());
}
}
}
程序運行結果: